How Developers Can Boost Their Debugging Abilities By Gustavo Woltmann
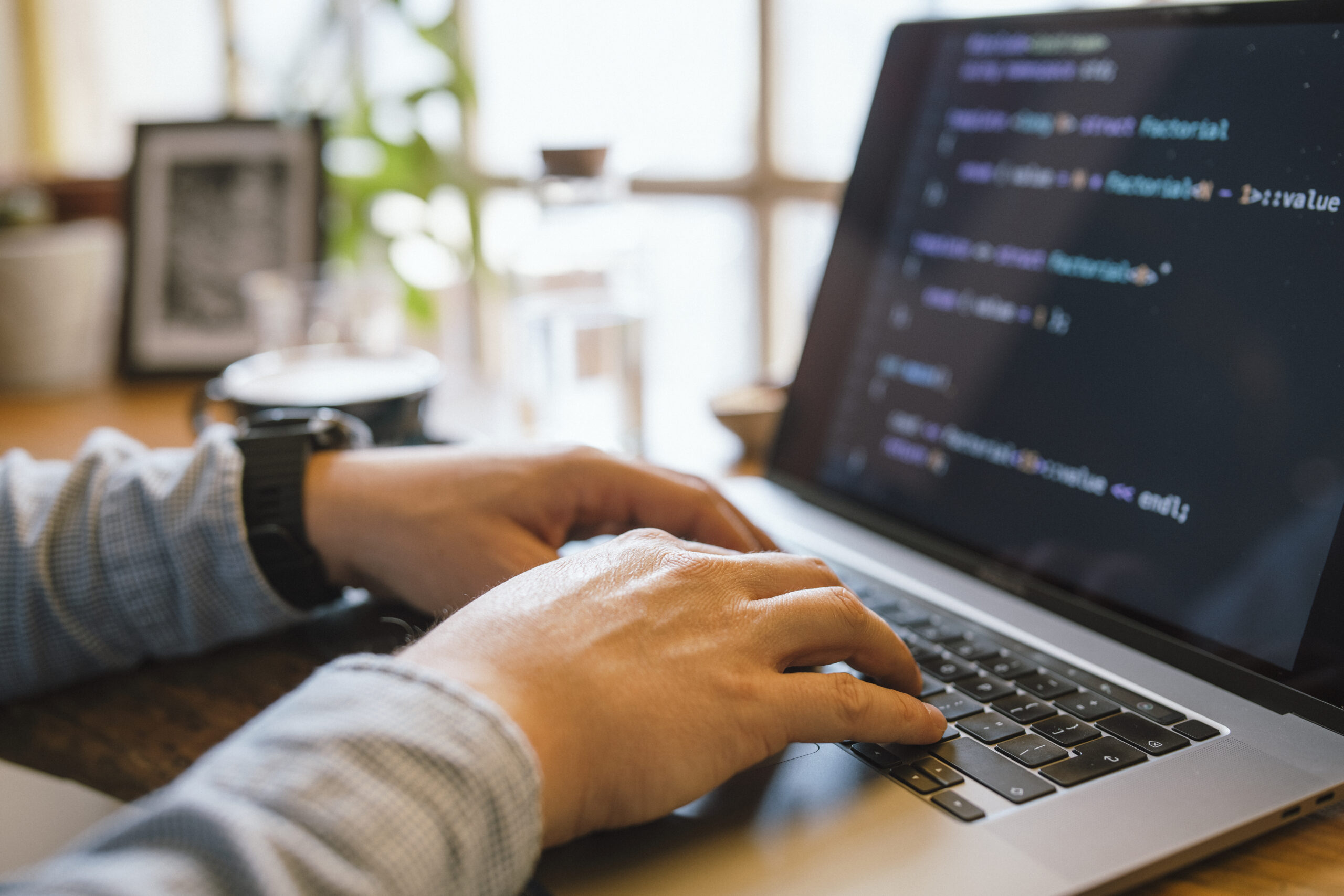
Debugging is One of the more necessary — yet usually neglected — techniques inside of a developer’s toolkit. It's not pretty much correcting damaged code; it’s about understanding how and why things go Incorrect, and Understanding to Assume methodically to unravel complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging expertise can preserve hrs of stress and substantially increase your productiveness. Here i will discuss quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Equipment
Among the quickest means builders can elevate their debugging capabilities is by mastering the instruments they use every single day. Although creating code is one part of enhancement, knowing ways to connect with it proficiently for the duration of execution is equally important. Modern-day development environments appear equipped with powerful debugging abilities — but several builders only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Progress Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step by code line by line, and in many cases modify code within the fly. When utilized effectively, they Allow you to notice precisely how your code behaves throughout execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion builders. They allow you to inspect the DOM, keep an eye on community requests, check out authentic-time overall performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can turn annoying UI issues into manageable jobs.
For backend or program-stage developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control in excess of operating processes and memory administration. Learning these resources could have a steeper Discovering curve but pays off when debugging overall performance challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation control methods like Git to grasp code history, discover the exact second bugs have been launched, and isolate problematic improvements.
In the end, mastering your equipment suggests likely further than default configurations and shortcuts — it’s about developing an intimate familiarity with your progress ecosystem so that when problems come up, you’re not misplaced at midnight. The greater you already know your instruments, the greater time you may commit fixing the actual challenge in lieu of fumbling by the method.
Reproduce the challenge
The most vital — and often neglected — techniques in powerful debugging is reproducing the challenge. Just before jumping into your code or creating guesses, builders have to have to make a steady atmosphere or state of affairs wherever the bug reliably appears. Without having reproducibility, fixing a bug results in being a video game of possibility, frequently bringing about squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What actions resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater element you've got, the easier it will become to isolate the exact disorders beneath which the bug takes place.
As you’ve collected more than enough details, try to recreate the challenge in your local setting. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions concerned. These tests not simply help expose the issue and also prevent regressions Later on.
From time to time, The problem may very well be atmosphere-distinct — it'd occur only on specific running units, browsers, or underneath individual configurations. Utilizing equipment like Digital devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating these bugs.
Reproducing the problem isn’t only a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You may use your debugging tools more effectively, test possible fixes safely, and communicate much more Obviously using your crew or end users. It turns an abstract grievance into a concrete challenge — Which’s in which developers thrive.
Study and Realize the Error Messages
Error messages are often the most precious clues a developer has when one thing goes Improper. As opposed to seeing them as irritating interruptions, builders really should study to take care of mistake messages as direct communications in the system. They normally inform you just what happened, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Get started by looking at the concept carefully and in comprehensive. A lot of developers, specially when beneath time pressure, look at the very first line and right away start building assumptions. But deeper during the error stack or logs may perhaps lie the real root trigger. Don’t just copy and paste mistake messages into search engines like yahoo — read and recognize them initial.
Split the error down into areas. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it position to a specific file and line variety? What module or function induced it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re applying. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and learning to recognize these can considerably speed up your debugging approach.
Some faults are obscure or generic, As well as in those circumstances, it’s important to look at the context by which the error transpired. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede more substantial problems and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint problems more quickly, lessen debugging time, and turn into a additional successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most powerful equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, supporting you fully grasp what’s occurring underneath the hood without needing to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Common logging levels include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info during advancement, Information for general situations (like thriving start off-ups), WARN for possible issues that don’t crack the applying, ERROR for real problems, and Lethal once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your program. Concentrate on vital functions, state variations, enter/output values, and significant choice details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and performance names, so it’s easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-considered-out logging method, you may decrease the time it will require to identify problems, get further visibility into your applications, and improve the Total maintainability and trustworthiness of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers ought to solution the process like a detective solving a mystery. This frame of mind can help stop working elaborate concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering proof. Think about the indications of the condition: mistake messages, incorrect output, or efficiency troubles. The same as a detective surveys against the law scene, accumulate just as much suitable information and facts as you can without having jumping to conclusions. Use logs, examination scenarios, and consumer studies to piece collectively a transparent photo of what’s occurring.
Upcoming, type hypotheses. Inquire your self: What might be creating this behavior? Have any modifications not too long ago been manufactured on the codebase? Has this concern transpired ahead of below comparable instances? The target is usually to narrow down possibilities and detect opportunity culprits.
Then, take a look at your theories systematically. Seek to recreate the situation within a managed ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay near interest to compact aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-just one error, or maybe a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of fully knowledge it. Short-term fixes may perhaps hide the true problem, just for it to resurface later.
And finally, keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and enable Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in sophisticated devices.
Generate Tests
Creating assessments is among the simplest tips on how to enhance your debugging expertise and Over-all enhancement efficiency. Exams not merely enable capture bugs early but will also function a security Web that gives you self-confidence when creating adjustments towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly where and when a problem occurs.
Start with device tests, which concentrate on person functions or modules. These small, isolated tests can quickly expose whether a selected bit of logic is Performing as predicted. Any time a exam fails, you promptly know wherever to seem, drastically lowering the time spent debugging. Device assessments are Specifically helpful for catching regression bugs—concerns that reappear following previously remaining fastened.
Following, integrate integration tests and close-to-conclusion exams into your workflow. These help make sure a variety of portions of your application work alongside one another easily. They’re especially practical for catching bugs that arise in complicated systems with many elements or products and services interacting. If anything breaks, your assessments can tell you which Component of the pipeline failed and less than what situations.
Crafting exams also forces you to definitely Feel critically about your code. To test a aspect appropriately, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and less bugs.
When debugging a concern, writing a failing examination that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to concentrate on repairing the bug and enjoy your test move when The problem is fixed. This method makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the discouraging guessing game into a structured and predictable approach—serving to you capture much more bugs, more quickly and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your intellect, cut down frustration, and often see The difficulty from the new standpoint.
If you're much too near the code for much too lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just hours earlier. In this point out, your Mind gets considerably less productive at trouble-fixing. A short walk, a espresso crack, or maybe switching check here to a distinct activity for 10–quarter-hour can refresh your aim. Quite a few developers report locating the root of a dilemma when they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting down in front of a screen, mentally trapped, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Electricity as well as a clearer state of mind. You may perhaps quickly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
If you’re caught, a great general guideline will be to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind space to breathe, enhances your standpoint, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Just about every bug you come upon is more than just A brief setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing precious for those who take the time to reflect and evaluate what went Mistaken.
Start out by asking your self several essential issues when the bug is fixed: What prompted it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring issues or popular faults—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from annoyance to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. In any case, some of the best developers are usually not the ones who generate excellent code, but individuals that continually learn from their problems.
In the end, Every single bug you fix adds a completely new layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear away a smarter, additional able developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Whatever you do.